Rust and Python
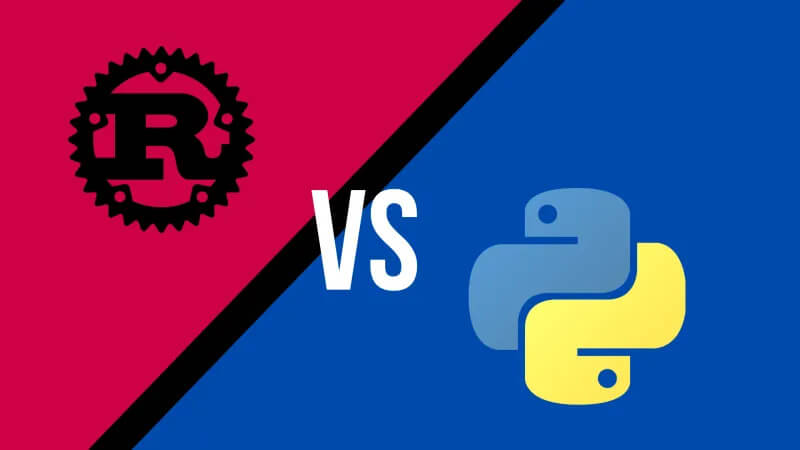
Rust and Python are both general-purpose programming languages, but they have different strengths and weaknesses.
- Rust is a systems programming language that is designed to be safe, fast, and expressive. It is compiled to native machine code, which makes it very efficient. Rust also has a strong focus on memory safety, which can help to prevent bugs and crashes.
- Python is an interpreted language that is designed to be easy to learn and use. It is often used for web development, data science, and machine learning. Python has a large and active community, and there are many libraries and frameworks available for it.
Which language is better?
The best language for you will depend on your specific needs and goals. If you need a language that is fast, safe, and memory-efficient, then Rust is a good choice. If you need a language that is easy to learn and use, and has a large community, then Python is a good choice.
Some examples of Rust and Python code
Rust
|
|
This Rust code defines a function called main()
, which increments the value of the variable x
by 1 and then prints the value of x
to the console.
Python
|
|
This Python code also defines a function called main()
, which increments the value of the variable x
by 1 and then prints the value of x
to the console. However, Python code is interpreted, not compiled, so the main()
function is not called until the end of the file.
As you can see, the Rust code is more concise and efficient than the Python code. However, the Python code is easier to read and understand.
Table that summarizes the key differences between Rust and Python
Some specific examples of where Rust and Python are commonly used
Rust is used for:
- Systems programming, such as operating systems and embedded systems.
- High-performance computing, such as game engines and scientific computing.
- Security-critical applications, such as web browsers and cryptography.
Python is used for:
- Web development, such as Django and Flask frameworks.
- Data science, such as NumPy and Pandas libraries.
- Machine learning, such as TensorFlow and PyTorch frameworks.
- General-purpose scripting, such as automating tasks and writing small programs.
Ultimately, the best way to decide which language is right for you is to try both of them and see which one you prefer.
Sharing is caring!